a better developer experience makes a better web
You could think about the web as two distinct worlds. The first of which is large and blasé in the face of change. Most of the web is in this category. Jquery and Wordpress are some of the most popular technologies here.
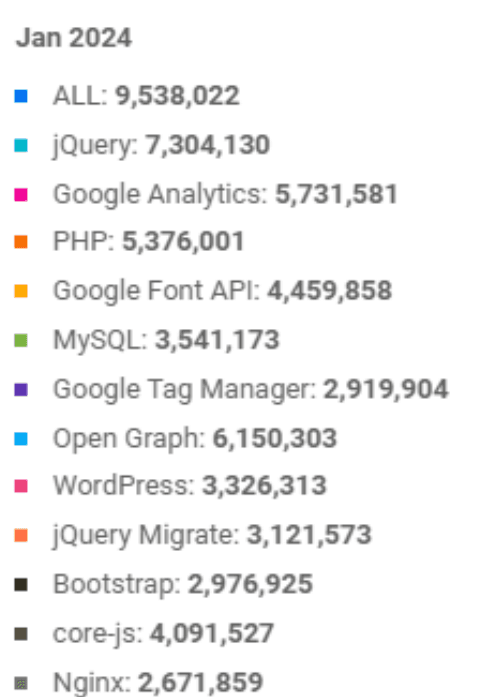
Then there is the other; the one where React is the king. Angular, Vue, Svelte, Solid, and Preact are the other top UI frameworks and more websites are built with React than all of them combined. This part of the web is distinguished and ambitious. Some of the most popular websites in history rely on UI frameworks. Netflix and AirBnB are built on React; Forbes on Angular; Gitlab on Vue, the list goes on.
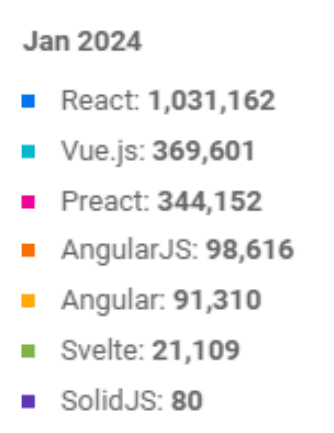
Subservient to the royalty of UI frameworks are a plethora of important web technologies. Implementations of bundling, routing, hydration, server rendering and many other robust features are rounded up by this world’s most capable royal subject; the web-framework, sometimes referred to as a meta-framework for clarity.
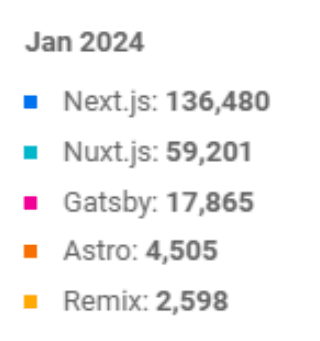
A lot of the reason why the web here feels fun to iterate on is because UI frameworks handle updates; and meta-frameworks fill in all the gaps necessary for a production ready application.
As React led the way on handling UI updates, it now vocalizes a new objective. A full stack architecture vision that claims we ought to navigate the server client boundary in a way native to the UI framework.
This vision has been met with pushback.
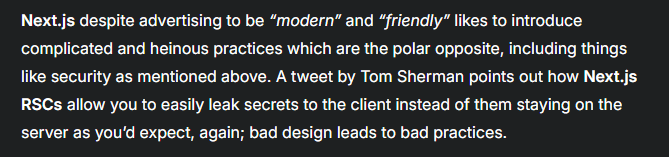
An author of a personal blog writes that Nextjs is heinous, as shown by server actions
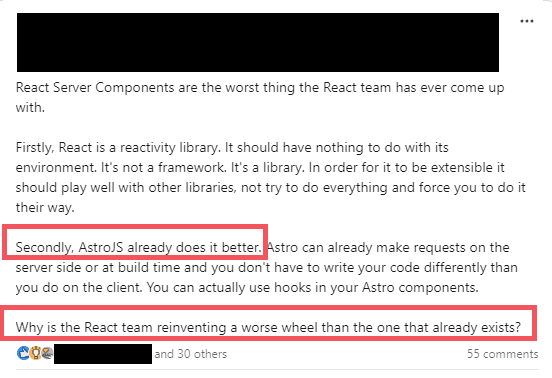
A Linkedin poster claims that Astro makes RSC pointless
The security issues with server actions have been raised as a criticism against RSC as a whole. Current documentation appropriately insists that server actions should be treated as public APIs – using them inappropriately is hardly grounds to critique the architecture as a whole.
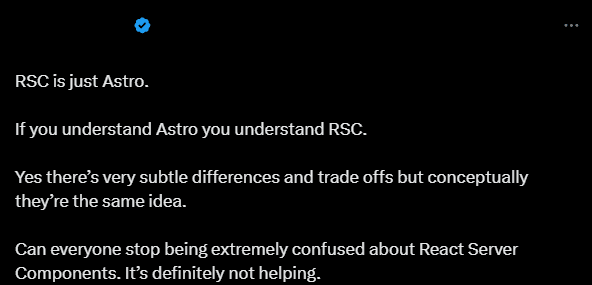
A dev on twitter accepts RSC to address the same issue as Astro

A well known creator puts it all together for us, emphasizing the UI native aspects of RSC in contrast to Astro.
Claims that meta-frameworks like Astro completely disqualify the React team's vision represent a reliable voice in favor of convention. In this particular case, it would be fair to contextualize these concerns as an opposition to UI frameworks having specifications for how to cross the client server boundary.
It is far from the first time React has been the target of scathing criticism regarding authority. When React was first introduced to the public, it was widely reprimanded for shipping a templating syntax that breached separations of concerns by combining XML and Javascript. In the end, the developer experience that came from this declarative syntax proved to be almost unanimously loved.
Though the true point of contention is in the division of authority, it has to be said that if any entity in the web ecosystem deserves the benefit of doubt when defying convention, it would be the team behind React.
For now, I can’t help but wonder if the same foundational elements of skepticism that vehemently opposed JSX in 2013 reemerge to be at odds with the new vision for React.
In the landscape where UI runtimes are designed with no expectation to opine outside of the browser, Astro's UI agnostic approach is a masterpiece. But we can now begin to see glimpses of how deferring to the UI's specification makes the web more fun to develop for.
Concretely, the leading UI framework shows us a vision for the future through streaming at the component level and abstractions over authoritative state mutations.
Streaming is necessary for many demanding web applications. Implementing it through React specification makes it a matter of wrapping your asynchronous component inside of Suspense. Astro makes it a matter of stepping in and out of the UI framework.
---
import Layout from "../layouts/Layout.astro;
import ServerComponent from "../components/ServerComponent.astro;
import PostFeed from "../components/PostFeed.astro;
---
<Layout title ="Welcome to Astro.">
<main>
<p class="spinner">Loading feed...</p>
<ServerComponent>
<PostFeed/>
</ServerComponent>
</main>
</Layout>
<script>
const serverComponent = document.querySelector(".serverComponent");
const loading = document.querySelector(".loading");
if (serverComponent && loading)
{
serverComponent.style.display = "block";
loading.style.display = "none";
}
</script>
Implementating loading state for a streamed component through Astro
import { Suspense } from "react";
import { PostFeed } from "./components/PostFeed";
export default function Home() {
return (
<div>
<Suspense fallback = {<p>Loading feed..</p>}>
<PostFeed/>
</Suspense>
</div>
);
}
The app router's Suspense support does the same, but better, in three lines
When streaming is only for meta-framework components, we lose both precision and convenience.
Server actions represent a granular approach to fine design. They feel great, until they don't work for you -- they don't [naturally] respond in parallel since they're meant to mutate authoritative server state, but they feel great to write and call.
The traditional way to perform these mutations is consuming a post request. First an endpoint is defined.
import type { NextApiRequest, NextApiReponse } from 'next';
export default async function handler(
req: NextApiRequest,
res: NextApiResponse
)
{
if (req.method !== 'POST'){
return res.status(405).json({message: 'Not Allowed'})
}
try {
const { customerId, amount, status } = req.body
// Server mutation
res.status(200).json({ message: 'Success' })
}
catch (error) {
res.status(500).json({ message: 'Error' })
}
And then it is consumed.
async function handleSubmit(event: FormEvent<HTMLFormElement>) {
event.preventDefault();
const form = event.currentTarget;
const formData = new FormData(form);
const rawFormData = {
customerId: formData.get('customerId'),
amount: formData.get('amount'),
status: formData.get('status'),
};
try {
const response = await fetch('api/create-invoice', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
// Should perform Authorization here
},
body: JSON.stringify(rawFormData),
});
if (!response.ok) {
throw new Error('Failed to create invoice');
}
} catch (err) {
console.error('Error creating invoice', err);
}
}
return (
<form onSubmit={handleSubmit}>
{/* Form fields go here */}
</form>
);
The traditional way to mutate authoritative state
Server actions put these two phases together into one.
async function createInvoice(formData: FormData) {
'use server';
// Should Authorize here
const rawFormData = {
customerId: formData.get('customerId'),
amount: formData.get('amount'),
status: formData.get('status'),
};
try {
// Server mutation
} catch (err) {
throw new Error('Error creating invoice');
}
}
return (
<form action={createInvoice}>
{/* Form fields go here */}
</form>
);
The RSC way
While it's not a revolutionary change, it's a promising glimspe into a future where the web develops even faster.
React’s popularity shows that the UI framework is at the helm of DX. At the same time, it represents parallels with the older web that is nothing if not afraid of change. The key difference is that when React is in the seat of power, their team has actively pursued change.
React’s new vision is a strong bet to make the web better; while RSC implementation details can be confusing, using them can be the most fun, and fast, way to build highly capable user interfaces. The React team’s bet is on crossing boundaries to improve DX and push the web to be even more robust. It should be fairly criticized, but it should first be applauded. Whether or not it was a sign of arrogance for the king to have ruled will only be determined by how the web is pushed by the bleeding edge.
Thanks for reading!